Creating shapesΒΆ
There are 3 basic shape nodes: Triangle, Quad and Circle. It is also possible to create custom geometries with the Geometry node.
Rendering a triangle
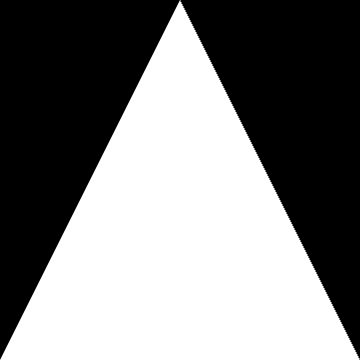
import pynopegl as ngl
@ngl.scene()
def triangle(cfg: ngl.SceneCfg):
cfg.aspect_ratio = (1, 1)
return ngl.RenderColor(geometry=ngl.Triangle())
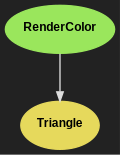
Rendering a quadrilateral
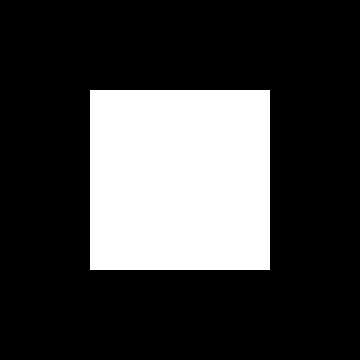
import pynopegl as ngl
@ngl.scene()
def quad(cfg: ngl.SceneCfg):
cfg.aspect_ratio = (1, 1)
return ngl.RenderColor(geometry=ngl.Quad())
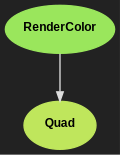
Rendering a circle with 64 points
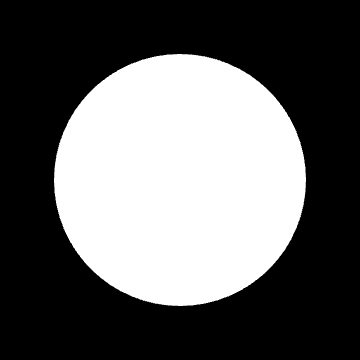
import pynopegl as ngl
@ngl.scene()
def circle(cfg: ngl.SceneCfg):
cfg.aspect_ratio = (1, 1)
return ngl.RenderColor(geometry=ngl.Circle(radius=0.7, npoints=64))
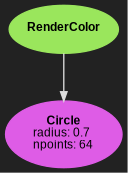
Rendering a custom geometry composed of 4 random points
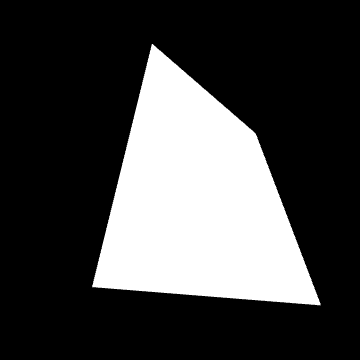
import array
import pynopegl as ngl
@ngl.scene()
def geometry(cfg: ngl.SceneCfg):
cfg.aspect_ratio = (1, 1)
p0 = (cfg.rng.uniform(-1, 0), cfg.rng.uniform(0, 1), 0)
p1 = (cfg.rng.uniform(0, 1), cfg.rng.uniform(0, 1), 0)
p2 = (cfg.rng.uniform(-1, 0), cfg.rng.uniform(-1, 0), 0)
p3 = (cfg.rng.uniform(0, 1), cfg.rng.uniform(-1, 0), 0)
vertices = array.array("f", p0 + p1 + p2 + p3)
uvcoords = array.array("f", p0[:2] + p1[:2] + p2[:2] + p3[:2])
geometry = ngl.Geometry(
vertices=ngl.BufferVec3(data=vertices),
uvcoords=ngl.BufferVec2(data=uvcoords),
topology="triangle_strip",
)
return ngl.RenderColor(geometry=geometry)
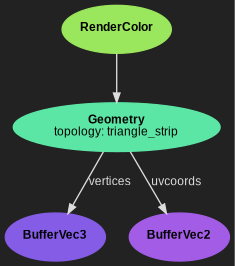